API access: Become a QR code generator
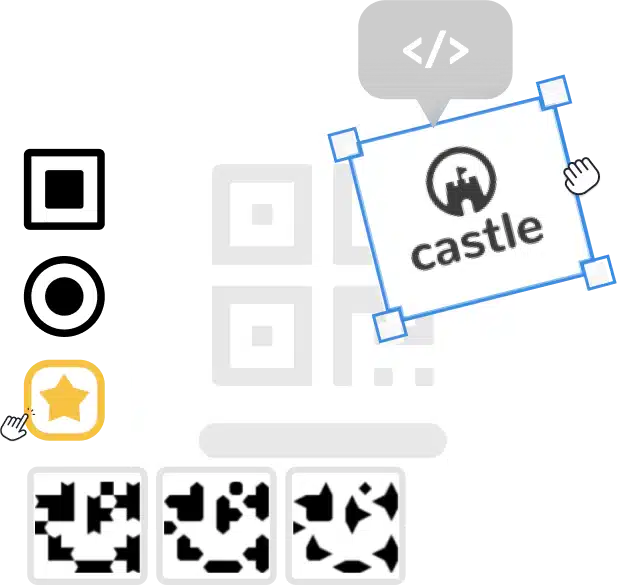
But first, what’s a QR code API?
For the rest of us, with an API you can integrate different programs or apps. An API is an application programming interface that connects one system to another generating interactions between them. In our case we offer both dynamic and static QR code APIs.
Uses vary from bulk QR codes generation, to QR code with specific images or logos, QR codes for business cards, coupons, and everything you can imagine!
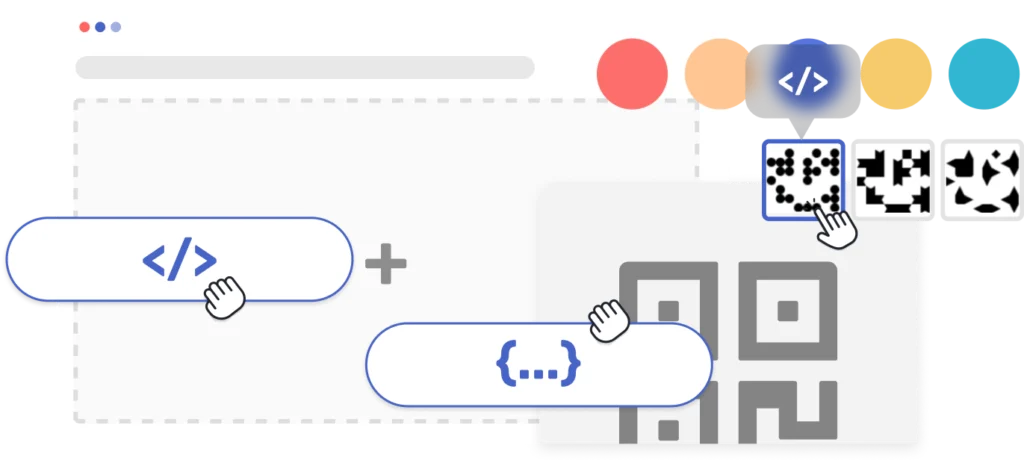
Let’s take a look at some examples of how the API can help your business or campaigns
Automation made easy
Our API was built to make your QR generation process smooth and effective. You can easily integrate our QR code generator with your iOS, Android systems or workflows.
Customization at scale
Massive creation with your brand guidelines it’s a must for us. That’s why with the API you can generate QR codes with your logo and brand colors!
Built for every need
Use it for employee IDs, digital business cards, generate tons of coupons for your customers, share documentation, everything your business needs. We have it or we create it 🙂
Just need to follow 3 simple steps
1 . API to create Dynamic QR codes
Go through the documentation for the v1.0 of the QR Code KIT dynamic QR code API. In order to access all the features provided by this API, you should request an API key at info@qrcodekit.com.
2 . Authentication
3 . QR codes
QR code API for QR codes creation
QR code API for basic black & white
Endpoint: https://api.uqr.me/api/1.0/
dynamicsqr/{{projectId}}/qrcode/
Headers:
Authorization: “Token ”
Content Type: “application/json”
Method: POST
Body:
{
"qr_type": "url",
"name": "QR Name",
"fields_data": {
"url": "https://qrcodekit.com",
"title": "My QR Title"
},
"attributes": {
"color": "#000000",
"background_color": "#FFFFFF"
}
}
Code examples
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => “https://api.uqr.me//api/1.0/dynamicsqr/{{projectId}}/qrcode/”,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => “”,
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => “POST”,
CURLOPT_POSTFIELDS =>”{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://qrcodekit.com\”\n\t},\n\t\”attributes\”: {\n\t\t\”color\”: \”#000000\”,\n\t\t\”background_color\”: \”#FFFFFF\”\n\t}\n}”,
CURLOPT_HTTPHEADER => array(
“Content-Type: application/json”,
“Authorization: Token {{YOUR API KEY}}”
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
curl –location –request POST ‘https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/’ \
–header ‘Content-Type: application/json’ \
–data-raw ‘{
“qr_type”: “url”,
“name”: “QR Name”,
“fields_data”: {
“url”: “https://qrcodekit.com”
},
“attributes”: {
“color”: “#000000”,
“background_color”: “#FFFFFF”
}
}’
var https = require(‘follow-redirects’).https;
var fs = require(‘fs’);
var options = {
‘method’: ‘POST’,
‘hostname’: ‘{{domain}}’,
‘path’: ‘https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/’,
‘headers’: {
‘Content-Type’: ‘application/json’
},
‘maxRedirects’: 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on(“data”, function (chunk) {
chunks.push(chunk);
});
res.on(“end”, function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on(“error”, function (error) {
console.error(error);
});
});
var postData = JSON.stringify({“qr_type”:”url”,”name”:”QR Name”,”fields_data”:{“url”:”https://qrcodekit.com”},”attributes”:
{“color”:”#000000″,”background_color”:”#FFFFFF”}});
req.write(postData);
req.end();
require “uri”
require “net/http”
url = URI(“https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/”)
http = Net::HTTP.new(url.host, url.port);
request = Net::HTTP::Post.new(url)
request[“Content-Type”] = “application/json”
request.body = “{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://qrcodekit.com”\n\t},\n\t\”attributes\”: {\n\t\t\”color\”: \”#000000\”,\n\t\t\”background_color\”: \”#FFFFFF\”\n\t}\n}”
response = http.request(request)
puts response.read_body
var client = new RestClient(“https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/”);
client.Timeout = -1;
var request = new RestRequest(Method.POST);
request.AddHeader(“Content-Type”, “application/json”);
request.AddParameter(“application/json”, “{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”:
{\n\t\t\”url\”: \”https://qrcodekit.com\”\n\t},\n\t\”attributes\”: {\n\t\t\”color\”: \”#000000\”,\n\t\t\”background_color\”: \”#FFFFFF\”\n\t}\n}”, ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Console.WriteLine(response.Content);
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse(“application/json”);
RequestBody body = RequestBody.create(mediaType, “{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://qrcodekit.com\”\n\t},\n\t\”attributes\”: {\n\t\t\”color\”: \”#000000\”,\n\t\t\”background_color\”: \”#FFFFFF\”}\n}”);
Request request = new Request.Builder()
.url(“https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/”)
.method(“POST”, body)
.addHeader(“Content-Type”, “application/json”)
.build();
Response response = client.newCall(request).execute();
RESPONSE:
{
'url' => ‘https://qrcodekit.to/XXXX,
'qr_code_image' => 'https://app.qrcodekit.com/qrs/XXXXXXXX.svg'
}
QR code API for colored QR codes
Endpoint: https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/
Headers:
Authorization: “Token ”
Content Type: “application/json”
Method: POST
Body:
{
"qr_type": "url",
"name": "QR Name",
"fields_data": {
"url": "https://qrcodekit.com",
"title": "My QR Title"
},
"attributes": {
"color": "#4663C6",
"background_color": "#F7F7F7"
"logo_image": "https://qrcodekitcdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png"
}
}
Code examples
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => “https://api.uqr.me//api/1.0/dynamicsqr/{{projectId}}/qrcode/”,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => “”,
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => “POST”,
CURLOPT_POSTFIELDS =>”{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://qrcodekit.com\”\n\t},\n\t\”attributes\”: {\n\t\t\”color\”: \”#4663C6\”,\n\t\t\”background_color\”: \”#F7F7F7\”\n\t}\n}”,
CURLOPT_HTTPHEADER => array(
“Content-Type: application/json”,
“Authorization: Token {{YOUR API KEY}}”
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
curl –location –request POST ‘https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/’ \
–header ‘Content-Type: application/json’ \
–data-raw ‘{
“qr_type”: “url”,
“name”: “QR Name”,
“fields_data”: {
“url”: “https://qrcodekit.com”
},
“attributes”: {
“color”: “#4663C6”,
“background_color”: “#F7F7F7”
}
}’
var https = require(‘follow-redirects’).https;
var fs = require(‘fs’);
var options = {
‘method’: ‘POST’,
‘hostname’: ‘{{domain}}’,
‘path’: ‘https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/’,
‘headers’: {
‘Content-Type’: ‘application/json’
},
‘maxRedirects’: 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on(“data”, function (chunk) {
chunks.push(chunk);
});
res.on(“end”, function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on(“error”, function (error) {
console.error(error);
});
});
var postData = JSON.stringify({“qr_type”:”url”,”name”:”QR Name”,”fields_data”:{“url”:”https://qrcodekit.com”},”attributes”:
{“color”:”#4663C6″,”background_color”:”#F7F7F7″}});
req.write(postData);
req.end();
require “uri”
require “net/http”
url = URI(“https://api.uqr.me.com/api/1.0/dynamicsqr/{{projectId}}/qrcode/”)
http = Net::HTTP.new(url.host, url.port);
request = Net::HTTP::Post.new(url)
request[“Content-Type”] = “application/json”
request.body = “{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://qrcodekit.com”\n\t},\n\t\”attributes\”: {\n\t\t\”color\”: \”#4663C6\”,\n\t\t\”background_color\”: \”#F7F7F7\”\n\t}\n}”
response = http.request(request)
puts response.read_body
var client = new RestClient(“https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/”);
client.Timeout = -1;
var request = new RestRequest(Method.POST);
request.AddHeader(“Content-Type”, “application/json”);
request.AddParameter(“application/json”, “{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”:
{\n\t\t\”url\”: \”https://qrcodekit.com\”\n\t},\n\t\”attributes\”: {\n\t\t\”color\”: \”#4663C6\”,\n\t\t\”background_color\”: \”#F7F7F7\”\n\t}\n}”, ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Console.WriteLine(response.Content);
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse(“application/json”);
RequestBody body = RequestBody.create(mediaType, “{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://qrcodekit.com\”\n\t},\n\t\”attributes\”: {\n\t\t\”color\”: \”#4663C6\”,\n\t\t\”background_color\”: \”#F7F7F7\”}\n}”);
Request request = new Request.Builder()
.url(“https://api.uqr.me.com/api/1.0/dynamicsqr/{{projectId}}/qrcode/”)
.method(“POST”, body)
.addHeader(“Content-Type”, “application/json”)
.build();
Response response = client.newCall(request).execute();
RESPONSE:
{
'url' => ‘https://qrcodekit.to/XXXX,
'qr_code_image' => 'https://app.qrcodekit.com/qrs/XXXXXXXX.svg'
}
QR code API to create QR codes with logo
Endpoint: https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/
Headers:
Authorization: “Token ”
Content Type: “application/json”
Method: POST
Body:
{
"qr_type": "url",
"name": "QR Name",
"fields_data": {
"url": "https://qrcodekit.com",
"title": "My QR Title"
},
"attributes": {
"color": "#E5FCC2",
"background_color": "#594f4f"
"logo_image": "https://qrcodekitcdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png"
}
}
Code examples
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => “https://api.uqr.me//api/1.0/dynamicsqr/{{projectId}}/qrcode/”,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => “”,
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => “POST”,
CURLOPT_POSTFIELDS =>”{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”: {\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://qrcodekit.com\”\n\t},\n\t\”attributes\”: {\n\t\t\”color\”: \”#4663C6\”,\n\t\t\”background_color\”: \”#F7F7F7\”,\n\t\t\”logo_image\”: \”https://qrcodekitmecdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png\”\n\t}\n}”,
CURLOPT_HTTPHEADER => array(
“Content-Type: application/json”,
“Authorization: Token {{YOUR API KEY}}”
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
curl –location –request POST ‘https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/’ \
–header ‘Content-Type: application/json’ \
–data-raw ‘{
“qr_type”: “url”,
“name”: “QR Name”,
“fields_data”: {
“url”: “https://qrcodekit.com”
},
“attributes”: {
“color”: “#4663C6”,
“background_color”: “#F7F7F7”
“logo_image”:”https://qrcodekitcdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png”
}
}’
var https = require(‘follow-redirects’).https;
var fs = require(‘fs’);
var options = {
‘method’: ‘POST’,
‘hostname’: ‘{{domain}}’,
‘path’: ‘https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/’,
‘headers’: {
‘Content-Type’: ‘application/json’
},
‘maxRedirects’: 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on(“data”, function (chunk) {
chunks.push(chunk);
});
res.on(“end”, function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on(“error”, function (error) {
console.error(error);
});
});
var postData = JSON.stringify({“qr_type”:”url”,”name”:”QR Name”,”fields_data”:{“url”:”https://qrcodekit.com”},”attributes”:
{“color”:”#4663C6″,”background_color”:”#F7F7F7,”logo_image”:”https://qrcodekitmecdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png”}});
req.write(postData);
req.end();
require “uri”
require “net/http”
url = URI(“https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/”)
http = Net::HTTP.new(url.host, url.port);
request = Net::HTTP::Post.new(url)
request[“Content-Type”] = “application/json”
request.body = “{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://qrcodekit.com”\n\t},\n\t\”attributes\”: {\n\t\t\”color\”: \”#4663C6\”,\n\t\t\”background_color\”: \”#F7F7F7\,\n\t\t\”logo_image\”: \”https://qrcodekitmecdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png\”\n\t}\n}”
response = http.request(request)
puts response.read_body
var client = new RestClient(“https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/”);
client.Timeout = -1;
var request = new RestRequest(Method.POST);
request.AddHeader(“Content-Type”, “application/json”);
request.AddParameter(“application/json”, “{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”:
{\n\t\t\”url\”: \”https://qrcodekit.com\”\n\t},\n\t\”attributes\”: {\n\t\t\”color\”: \”#4663C6\”,\n\t\t\”background_color\”: \”#F7F7F7\”\n\t}\n”logo_image\”: \”https://qrcodekitmecdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png\}”, ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Console.WriteLine(response.Content);
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse(“application/json”);
RequestBody body = RequestBody.create(mediaType, “{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR Name\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://qrcodekit.com\”\n\t},\n\t\”attributes\”: {\n\t\t\”color\”: \”#4663C6\”,\n\t\t\”background_color\”: \”#F7F7F7\,”\n\t\t\”logo_image\”: \”https://qrcodekitmecdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png\\n}”);
Request request = new Request.Builder()
.url(“https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/”)
.method(“POST”, body)
.addHeader(“Content-Type”, “application/json”)
.build();
Response response = client.newCall(request).execute();
RESPONSE:
{
'url' => ‘https://qrcodekit.to/XXXX,
'qr_code_image' => 'https://app.qrcodekit.com/qrs/XXXXXXXX.svg'
}
QR code API to create QR codes with advanced design and logo
Endpoint: https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/
Headers:
Authorization: “Token ”
Content Type: “application/json”
Method: POST
Body:
{
"qr_type": "url",
"name": "QR Name",
"fields_data": {
"url": "https://qrcodekit.com",
"title": "My QR Title"
},
"attributes": {
"body": "round",
"color": "#000000",
"background_color": "#ffffff",
"eye1": "frame1",
"eyeBall1": "ball1",
"eye1Color": "#ed5a4f",
"eyeBall1Color": "#ed5a4f",
"setEyesAllAtOnce": "true",
"errorCorrection": "3",
"logoPercent": "0.4"
"mode": "advanced",
"logo_image": "https://qrcodekitmecdn.s3.us-east- 2.amazonaws.com/u/16/16-24-logo.png""
}
}
Advanced QR code settings documentation
PARAMETER NAME
color
background_color
logo
logoPercent
errorCorrection
DEFAULT VALUE
#000000
#FFFFFF
No logo
0.2
0
DESCRIPTION
Foreground color in the format #RRGGBB
Bkg color in the format #RRGGBB
URL of the logo to include
Value from 0.2 to 1. It can make the QR unreadable. The value 1 is used with transparent PNGs so that the logo is not positioned in the center
Four levels of error correction are supported, with L being the least thorough and H the most comprehensive.
0 => L
1 => M
2 => Q
3 => H
Advanced options: They are used to modify the style and layout of the QR.
PARAMETER NAME
setEyesAllAtOnce
eye1
eye2
eye3
eyeBall1
eyeBall2
eyeBall3
eye1Color
eye2Color
eye3Color
body
hasGradient
gradientColor1
gradientColor2
gradientType
VALUE
true
frame0
frame0
frame0
ball0
ball0
ball0
#000000
#000000
#000000
square
false
#000000
#000000
linear
DESCRIPTION
If true, set all large squares with the same shape and color
Same options as eye1, only taken into account if setEyes
AllAtOnce is set to true
Same options as eye1, only taken into account if setEyes
AllAtOnce is set to true
Same options as eyeBall1, only taken into account if setEyes
AllAtOnce is set to true
Only taken into account if setEyes
AllAtOnce is set to true
Big square color in #RRGGBB format
AtOnce is false. Big square color in #RRGGBB format
AtOnce is false. Big square color in #RRGGBB format
If true, applies a gradient to the QR
First color of the gradient
Second color of the gradient
Typology of the gradient. The options are:
Code examples
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => “https://api.uqr.me//api/1.0/dynamicsqr/{{projectId}}/qrcode/”,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => “”,
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => “POST”,
CURLOPT_POSTFIELDS =>”{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR hecho con el API\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://www.google.com\”,\n\t\t\”title\”: \”Mi QR\”\n\t},\n\t\”attributes\”: {\n\”body\” : \”round\”,\n\”color\” : \”#4663C6\”,\n \”background_color\” : \”#F7F7F7\”,\n \”eye1\” : \”frame1\”,\n\”eyeBall1\” : \”ball1\”,\n\”eye1Color\” : \”#FE5B54\”,\n\”eyeBall1Color\” : \”#FE5B54\”,\n\”setEyesAllAtOnce\” : \”true\”,\n\”errorCorrection\” : \”3\”,\n\”logoPercent\” : \”0.4\”,\n\”mode\”: \”advanced\”,\n\”logo_image\”: \”https://qrcodekitmecdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png\”\n\t}\n}”,
CURLOPT_HTTPHEADER => array(
“Content-Type: application/json”,
“Authorization: Token {{YOUR API KEY}}”
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
curl –location –request POST ‘https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/’ \
–header ‘Content-Type: application/json’ \
–data-raw ‘{
“qr_type”: “url”,
“name”: “QR Name”,
“fields_data”: {
“url”: “https://qrcodekit.com”
},
“attributes”: {
“color”: “#4663C6”,
“background_color”: “#F7F7F7”,
“eye1”: “frame1”,
“eyeBall1”: “ball1”,
“eye1Color”: “#FE5B54”,
“eyeBall1Color”: “#FE5B54”,
“setEyesAllAtOnce”: “true”,
“errorCorrection”: “3”,
“logoPercent”: “0.4”,
“mode”: “advanced”,
“logo_image”:”https://qrcodekitmecdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png”
}
}’
var https = require(‘follow-redirects’).https;
var fs = require(‘fs’);
var options = {
‘method’: ‘POST’,
‘hostname’: ‘{{domain}}’,
‘path’: ‘https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/’,
‘headers’: {
‘Content-Type’: ‘application/json’
},
‘maxRedirects’: 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on(“data”, function (chunk) {
chunks.push(chunk);
});
res.on(“end”, function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on(“error”, function (error) {
console.error(error);
});
});
var postData = JSON.stringify({“qr_type”:”url”,”name”:”QR Name”,”fields_data”:{“url”:”https://www.google.com”},”attributes”:
{ “body” : “round”, “color” : “#4663C6”, “background_color” : “#F7F7F7”, “eye1” : “frame1”, “eyeBall1” : “ball1”, “eye1Color” : “#FE5B54”, “eyeBall1Color” : “#FE5B54”, “setEyesAllAtOnce” : “true”, “errorCorrection” : “3”, “logoPercent” : “0.4”, “mode”: “advanced”, “logo_image”: “https://qrcodekitmecdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png” } });
req.write(postData);
req.end();
require “uri”
require “net/http”
url = URI(“https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/”)
http = Net::HTTP.new(url.host, url.port);
request = Net::HTTP::Post.new(url)
request[“Content-Type”] = “application/json”
request.body = “{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR hecho con el API\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://www.google.com\”,\n\t\t\”title\”: \”Mi QR\”\n\t},\n\t\”attributes\”: {\n\”body\” : \”round\”,\n\”color\” : \”#4663C6\”,\n \”background_color\” : \”#F7F7F7\”,\n \”eye1\” : \”frame1\”,\n\”eyeBall1\” : \”ball1\”,\n\”eye1Color\” : \”#FE5B54\”,\n\”eyeBall1Color\” : \”#FE5B54\”,\n\”setEyesAllAtOnce\” : \”true\”,\n\”errorCorrection\” : \”3\”,\n\”logoPercent\” : \”0.4\”,\n\”mode\”: \”advanced\”,\n\”logo_image\”: \”https://qrcodekitmecdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png\”\n\t}\n}”
response = http.request(request)
puts response.read_body
var client = new RestClient(“https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/”);
client.Timeout = -1;
var request = new RestRequest(Method.POST);
request.AddHeader(“Content-Type”, “application/json”);
request.AddParameter(“application/json”, “{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR hecho con el API\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://www.google.com\”,\n\t\t\”title\”: \”Mi QR\”\n\t},\n\t\”attributes\”: {\n\”body\” : \”round\”,\n\”color\” : \”#4663C6\”,\n \”background_color\” : \”#F7F7F7\”,\n \”eye1\” : \”frame1\”,\n\”eyeBall1\” : \”ball1\”,\n\”eye1Color\” : \”#FE5B54\”,\n\”eyeBall1Color\” : \”#FE5B54\”,\n\”setEyesAllAtOnce\” : \”true\”,\n\”errorCorrection\” : \”3\”,\n\”logoPercent\” : \”0.4\”,\n\”mode\”: \”advanced\”,\n\”logo_image\”: \”https://qrcodekitmecdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png\”\n\t}\n}”, ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Console.WriteLine(response.Content);
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse(“application/json”);
RequestBody body = RequestBody.create(mediaType, “{\n\t\”qr_type\”: \”url\”,\n\t\”name\”: \”QR hecho con el API\”,\n\t\”fields_data\”: {\n\t\t\”url\”: \”https://www.google.com\”,\n\t\t\”title\”: \”Mi QR\”\n\t},\n\t\”attributes\”: {\n\”body\” : \”round\”,\n\”color\” : \”#4663C6\”,\n \”background_color\” : \”#F7F7F7\”,\n \”eye1\” : \”frame1\”,\n\”eyeBall1\” : \”ball1\”,\n\”eye1Color\” : \”#FE5B54\”,\n\”eyeBall1Color\” : \”#FE5B54\”,\n\”setEyesAllAtOnce\” : \”true\”,\n\”errorCorrection\” : \”3\”,\n\”logoPercent\” : \”0.4\”,\n\”mode\”: \”advanced\”,\n\”logo_image\”: \”https://qrcodekitmecdn.s3.us-east-2.amazonaws.com/u/16/16-24-logo.png\”\n\t}\n}”);
Request request = new Request.Builder()
.url(“https://api.uqr.me/api/1.0/dynamicsqr/{{projectId}}/qrcode/”)
.method(“POST”, body)
.addHeader(“Content-Type”, “application/json”)
.build();
Response response = client.newCall(request).execute();
RESPONSE:
{
'url' => ‘https://qrcodekit.to/XXXX,
'qr_code_image' => 'https://app.qrcodekit.com/qrs/XXXXXXXX.svg'
}